The Advanced Query Loop block (Kadence Blocks Pro)provides settings to create a custom query loop. However, sometimes, you need to alter the query beyond the capabilities of the Advanced Query Loop’s settings. In these cases, you can add a code snippet to alter the WordPress Query object. You can add PHP code to your site using a plugin like Code Snippets or your functions.php file (in a child theme).
If you want to modify the query arguments, you can make use of the ‘kadence_blocks_pro_query_loop_query_vars‘ filter. Here is an example that implements the filter.
Examples
add_filter( 'kadence_blocks_pro_query_loop_query_vars', function( $query, $ql_query_meta, $ql_id ) {
if ( $ql_id == 5283 ) {
$query['tax_query'] = array(
array(
'taxonomy' => 'category',
'field' => 'slug',
'terms' => 'sub',
)
);
}
return $query;
}, 10, 3 );
In the above code, the $ql_id is set to 5283. This is the post ID of the specific query you want to target. If you don’t provide the conditional “if” statement to target a query ID, all of your queries will share the arguments, which isn’t ideal. Among other ways, you can find the $ql_id by navigating to Kadence Blocks > All Queries, hovering over the edit link of your desired query, and looking at the bottom left corner of your browser window. Be sure to change the ID to suit your needs.
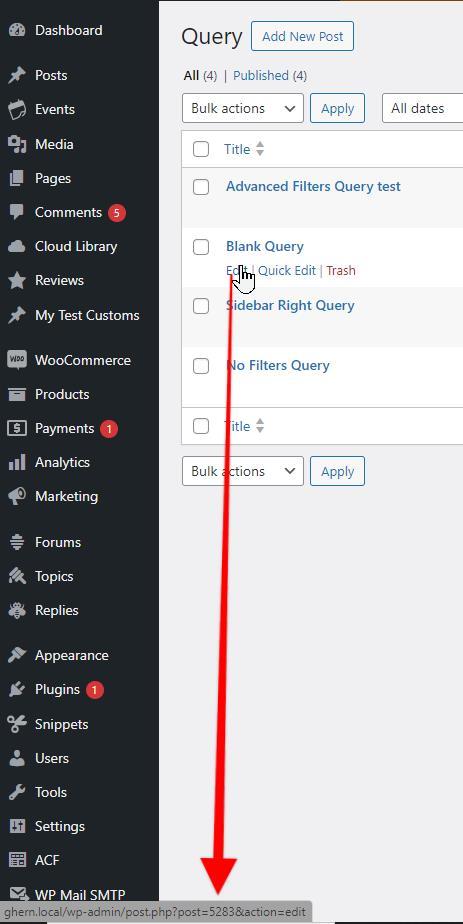
The example also uses the Simple Taxonomy Query provided by the WordPress Query documentation. Here are some additional examples that have been modified from the WordPress documentation.
Returns posts dated December 12, 2012
Source: https://developer.wordpress.org/reference/classes/wp_query/#date-parameters
add_filter( 'kadence_blocks_pro_query_loop_query_vars', function( $query, $ql_query_meta, $ql_id ) {
if ( $ql_id == 5283 ) {
$query['date_query'] = array(
array(
'year' => 2012,
'month' => 12,
'day' => 12,
),
);
}
return $query;
}, 10, 3 );
Display posts where the custom field key is ‘color’ and the custom field value IS ‘blue’:
Source: https://developer.wordpress.org/reference/classes/wp_query/#custom-field-post-meta-parameters
add_filter( 'kadence_blocks_pro_query_loop_query_vars', function( $query, $ql_query_meta, $ql_id ) {
if ( $ql_id == 5283 ) {
$query['meta_key'] = 'color';
$query['meta_value'] = 'blue';
$query['meta_compare'] = '=';
}
return $query;
}, 10, 3 );
Display posts with 25 comments or more:
Source: https://developer.wordpress.org/reference/classes/wp_query/#comment-parameters.
add_filter( 'kadence_blocks_pro_query_loop_query_vars', function( $query, $ql_query_meta, $ql_id ) {
if ( $ql_id == 5283 ) {
$query['post_type'] = 'post';
$query['comment_count'] = array(
'value' => 1,
'compare' => '>=',
);
}
return $query;
}, 10, 3 );
Display post that are not in a specific category:
Source: https://developer.wordpress.org/reference/classes/wp_query/#category-parameters
add_filter( 'kadence_blocks_pro_query_loop_query_vars', function( $query, $ql_query_meta, $ql_id ) {
if ( $ql_id == 5283 ) {
$query['category__not_in'] = 5;
}
return $query;
}, 10, 3 );